TL;DR:
AllTick API provides real-time and historical data for a wide range of markets, including:
– Hong Kong Stocks
– China Stocks
– U.S. Stocks
– Forex
– Crypto
– Commodities
Features:
Real-time & historical data available
Latency as low as 170ms
Account registration: https://alltick.co/en-US/register
API Docs: https://en.apis.alltick.co/
Github: https://github.com/alltick/
Actually, getting live forex data is pretty important these days. The market’s moving fast, and every second counts. And if you’re trading or analyzing forex, you know that having reliable, up-to-the-minute data can make a big difference. So, this guide is here to help you tap into AllTick’s Forex live API, which gives you just that—real-time and historical data with super low lag.
Why should you care? Well, there are lots of data providers out there, but AllTick’s got some big advantages. Fast, accurate, and easy to use. We’ll break down exactly what makes AllTick different and why it might be your best bet for getting the info you need, right when you need it.
Who’s behind this? Us. The AllTick team. We’ve spent years helping traders and financial platforms around the world access data they can trust. So, we know what traders need—and we’re here to make sure you can get the most out of our API.
And here’s the best part. By the time you finish this guide, you’ll know how to pull live forex data with AllTick, understand its standout features, and get hands-on with easy-to-follow code examples. It’s everything you need to get set up and stay ahead.
Key Features of AllTick’s for Forex Market Data API
So, what makes AllTick’s Forex API stand out? Let’s dive into the key features that can really give you the edge in your trading.
Real-time Data
First off, the real-time data is top-notch. When you pull forex data from AllTick, it’s not just fast—it’s lightning-fast. The data updates in real-time, so you’re always getting the freshest information. This matters a lot, especially if you’re into high-frequency trading or just want to stay ahead of the market.
Historical Data
AllTick also gives you access to historical data. This is perfect if you want to go back in time and test your strategies or just analyze market trends over the long haul. Whether you’re looking for minute-by-minute, hourly, or daily data, you’ve got it.
Low Latency
AllTick’s real-time data stream is delivered through WebSocket, and it has ultra-low latency—averaging only about 170 milliseconds. That means the data you receive is almost instant, allowing you to react to market changes without delay.
How to Create an Account on AllTick
Getting started with AllTick is super easy and doesn’t cost you a thing. Here’s how to create your account and get your hands on that sweet forex data.
Sign Up for an Account
Head over to the registration page here and fill in your details. The sign-up process is free, and no credit card info is required.
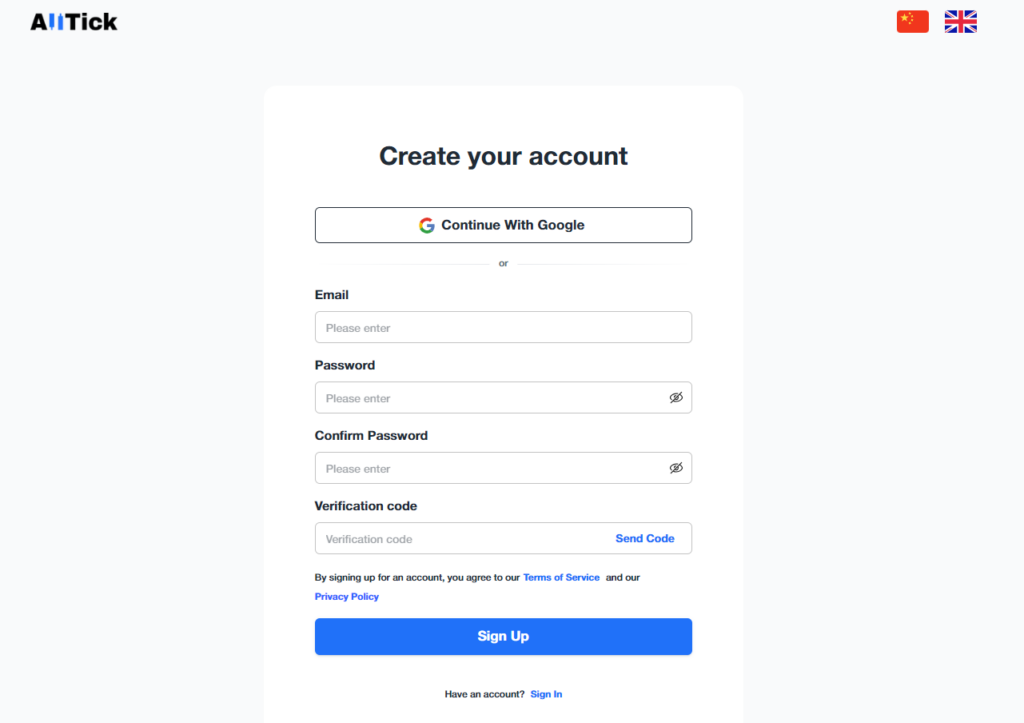
Access Your Dashboard & API Key
Once you complete the registration, you’ll be redirected to your account dashboard. Here, you’ll find your personal API key—this is what you’ll use every time you send a request via the API to access the forex data. Keep it safe!
That’s it! You’re ready to go. Just grab your API key and start integrating the data into your system. It’s quick, free, and easy—so you can focus on what matters most.
Comparing AllTick Plans
AllTick offers a variety of plans designed to suit different trading needs. Whether you’re just starting out or you’re a professional trader needing full access to data, there’s a plan that works for you. Let’s break down the differences between each plan so you can choose the one that best fits your requirements.
Here’s a side-by-side comparison of the plans:
Plan | Free | Basic | Premium | Pro | All HK Stocks | All CN Stocks | All US Stocks |
Monthly Price | 0 | 99USDT | 199USDT | 399USDT | 399USDT | 599USDT | 799USDT |
Product Available | 10 | 100 | 200 | 3000 | Full HK Stock Market Data | Full CN Stock Market Data | Full US Stock Market Data |
API Calls per Minute | 10 | 60 | 600 | 1200 | 1200 | 1200 | 1200 |
WebSockets | 1 | 1 | 3 | 10 | 10 | 10 | 10 |
Historical Data | 1 Year | 1 Year | 3 Years | 5 Years | 5 Years | 5 Years | 5 Years |
Last Price | ✅ | ✅ | ✅ | ✅ | ✅ | ✅ | ✅ |
End of Day Data | ✅ | ✅ | ✅ | ✅ | ✅ | ✅ | ✅ |
Real-time K-line | ❎ | ✅ | ✅ | ✅ | ✅ | ✅ | ✅ |
Order Book | ❎ | ✅ | ✅ | ✅ | ✅ | ✅ | ✅ |
Tick data | ❎ | ✅ | ✅ | ✅ | ✅ | ✅ | ✅ |
Now you can easily see which plan fits your needs based on the type of data, API calls, and features you require. Whether you’re just starting out or need a full range of data for professional trading, AllTick has a plan that’s right for you.
Get Forex Quotes Using Rest API
Here’s a code example for how to retrieve forex quotes using AllTick’s REST API. This script fetches kline data, depth tick data, and trade tick data for different market symbols. You’ll need to replace testtoken
with your actual API token in the URLs.
import time import requests import json # Extra headers for the API request test_headers = { 'Content-Type': 'application/json' } # Note: Replace "testtoken" in the URLs below with your actual API token. # Define the API endpoints for different types of data test_url1 = 'https://quote.tradeswitcher.com/quote-stock-b-api/kline?token=testtoken&query=%7B%22trace%22%20%3A%20%22python_http_test1%22%2C%22data%22%20%3A%20%7B%22code%22%20%3A%20%22700.HK%22%2C%22kline_type%22%20%3A%201%2C%22kline_timestamp_end%22%20%3A%200%2C%22query_kline_num%22%20%3A%202%2C%22adjust_type%3A%200%7D%7D' test_url2 = 'https://quote.tradeswitcher.com/quote-stock-b-api/depth-tick?token=testtoken&query=%7B%22trace%22%20%3A%20%22python_http_test2%22%2C%22data%22%20%3A%20%7B%22symbol_list%22%3A%20%5B%7B%22code%22%3A%20%22700.HK%22%7D%2C%7B%22code%22%3A%20%22UNH.US%22%7D%5D%7D%7D' test_url3 = 'https://quote.tradeswitcher.com/quote-stock-b-api/trade-tick?token=testtoken&query=%7B%22trace%22%20%3A%20%22python_http_test3%22%2C%22data%22%20%3A%20%7B%22symbol_list%22%3A%20%5B%7B%22code%22%3A%20%22700.HK%22%7D%2C%7B%22code%22%3A%20%22UNH.US%22%7D%5D%7D%7D' # Send the first API request to retrieve kline data resp1 = requests.get(url=test_url1, headers=test_headers) time.sleep(1) # Pause for a moment to avoid hitting the rate limit # Send the second API request to get depth tick data resp2 = requests.get(url=test_url2, headers=test_headers) time.sleep(1) # Send the third API request for trade tick data resp3 = requests.get(url=test_url3, headers=test_headers) # Print the decoded text returned by each request text1 = resp1.text print("Kline Data:", text1) text2 = resp2.text print("Depth Tick Data:", text2) text3 = resp3.text print("Trade Tick Data:", text3)
WebSockets
Here is a code example for connecting to AllTick’s WebSocket API, which streams real-time market data such as stock prices, market depth, and more. This script demonstrates how to set up a WebSocket connection, subscribe to specific data streams, and handle incoming messages.
import json import websocket # Ensure you have the websocket-client library installed: pip install websocket-client ''' # Special Note: # GitHub: https://github.com/alltick/realtime-forex-crypto-stock-tick-finance-websocket-api # Token Application: https://alltick.co # Replace "testtoken" in the URL below with your actual token. # Stock API address: # wss://quote.tradeswitcher.com/quote-stock-b-ws-api ''' class Feed(object): def __init__(self): self.url = 'wss://quote.tradeswitcher.com/quote-stock-b-ws-api?token=testtoken' # Replace with your actual WebSocket URL and token self.ws = None def on_open(self, ws): """ Callback executed when the WebSocket connection is opened. """ print('A new WebSocketApp is opened!') # Example subscription parameters sub_param = { "cmd_id": 22002, "seq_id": 123, "trace": "3baaa938-f92c-4a74-a228-fd49d5e2f8bc-1678419657806", # Unique trace identifier "data": { "symbol_list": [ { "code": "700.HK", # Hong Kong stock code "depth_level": 5, # Market depth level }, { "code": "UNH.US", # U.S. stock code "depth_level": 5, # Market depth level } ] } } # Send the subscription request to WebSocket sub_str = json.dumps(sub_param) ws.send(sub_str) print("Depth quotes subscribed!") def on_message(self, ws, message): """ Callback executed when a message is received. """ # Parse the received message and print it result = json.loads(message) # Safely parse the JSON message print(result) def on_error(self, ws, error): """ Callback executed when an error occurs. """ print(error) def on_close(self, ws, close_status_code, close_msg): """ Callback executed when the WebSocket connection is closed. """ print('The connection is closed!') def start(self): """ Initiates the WebSocket connection and starts the listener. """ self.ws = websocket.WebSocketApp( self.url, on_open=self.on_open, on_message=self.on_message, on_error=self.on_error, on_close=self.on_close, ) self.ws.run_forever() # Keeps the connection open and continuously listens for messages if __name__ == "__main__": feed = Feed() feed.start() # Start the WebSocket feed
Get Started with AllTick API Today!
Ready to take your trading or data analysis to the next level? With AllTick’s powerful real-time market data API, you can access a wealth of information for stocks, forex, crypto, commodities, and more. Whether you’re building a trading strategy or integrating market data into your platform, AllTick’s APIs and WebSocket feeds offer the speed, flexibility, and reliability you need.
Sign up today and start accessing real-time market data:
Create your account and get started with our free plan or explore our premium options for even more data coverage.
For full documentation, check out our API docs or visit our GitHub for code examples and integration guides.
Take the first step in harnessing the power of real-time data with AllTick!